I am talking about a ping pong ball, squash ball and a golf ball. But more on that later.
I hate being wrong, I really do. But what is even worse, is the feeling of being right when someone tells you otherwise. This is the story about how TypeScript came to the rescue in such a situation.
Background
It all started when I had a chat with a few colleagues, and we started talking about the upcoming summer party at work. Our boss had earlier suggested that we might gather in his garden. We thought it was a good idea, and considering that he also has some military background, someone suggested that we might drive there in Humvees. Or, maybe drop down from a C-130 Hercules. Or, maybe we could drive a Humvee off a C-130 from a flyover and land the car in the garden! More or less like these guys (if you haven't watched this video, you should).
I had only one concern, though, and that was the weight of the Humvees. They are quite heavy, and I thought the fall velocity might be too high (before we considered parachutes). So, I expressed my concern out loud, at which point the avalanche started.
Tom: What, you think that the object's weight affects fall velocity? It doesn't, that's just a myth!
Peter: Yeah, don't you know, like, Newton's laws of motion?
Me: But... What about air resistance?
Tom: No, that doesn't matter - haven't you seen that guy on YouTube who drops two balls simultaneously, and they hit the ground at the same time?
Peter: Yeah, that guy!
Me: But... But..! AIR RESISTANCE!
At this point, two things happened.
- I was shocked. These guys are smart people, and excellent programmers for whom I have great respect. So I started doubting myself, and was not sure whether they were just pulling my leg, or if I might be wrong.
- John overheard our argument, and wondered if we were all back in kindergarden. That concluded the conversation, and we went to a meeting.
Checking the facts
I obviously could not let this pass! So I decided to recap Newton's second law of motion and other necessary laws of physics to get my theory right. And then, being a software developer, code a simulator!
Theory of falling objects
First things first. Newton's second law of motion states in short that the thing that determines an object's acceleration is the sum of all forces on that object, divided by the object's mass.
On Earth, all objects are pulled towards Earth by gravity, g, with an acceleration of 9.81 m/s2. That means that you can calculate the fall velocity of an object as a function of time.
But this formula is only valid in vacuum... When things fall in stuff that is not a vacuum, there is something called drag affecting the equation. Stuff like water or air. This is the formula for the velocity of things that fall in stuff that's not vacuum.
So... Things get more complex. I think an explanation of all the formula components is in order now.
- g = acceleration due to gravity (9.81 m/s2)
- m = mass of the falling object
- ρ = density of the fluid through which the object is falling
- Cd = drag coefficient
- A = projected area of the object
As time elapses, the tanh part of the formula will converge to 1, thus the first part represents the object's terminal velocity, or "maximum speed when falling in air". With m placed in the fraction's numerator, there is a direct correlation between an object's mass and it's terminal velocity - in theory, heavier objects should have a higher terminal velocity, given that the size and shape are the same.
That guy on YouTube
I'm not sure if I found the video that was cited in the original conversation, but I found this: Which hits the ground first?
In the video, a basket ball and medicine ball are dropped simultaneously. They are more or less the same shape and size, but the medicine ball is significantly heavier. People on the street are asked which will hit the ground first. Their answers are diverse, but when they drop the two balls, they hit the ground at the same time. The explanation is that more force is applied by gravity on the heavier ball, but it also has more inertia, which is correct. This makes the two balls fall at the same speed. But does it? Really? Let's build a simulator!
Simulating falling objects
There are many ways to simulate physics with a computer. For this particular task, I wanted to use something that met the following requirements.
- Visualize the simulation - makes it easier to understand the simulation results.
- Web ready - accessible to anyone who might want to try it out.
- Easy to develop clean code
Traditionally, the closest match for these requirements was Flash or Silverlight, none of which I wanted to use. But I had just recently picked up TypeScript, which was still in beta at the time. With classes, interfaces and types built into the language, and combined with HTML5 <canvas>
, it would be the perfect tool for this task.
A class hierarchy of shapes and movements was the basic elements of my simulator. Here is the class that implements the formula for falling objects in air.
export class FreeFall implements IMovement {
speed: number;
airDensity: number;
dt: number;
constructor(initialSpeed: number, airDensity: number) {
this.speed = initialSpeed;
this.airDensity = airDensity;
this.dt = 0;
}
move(shape: IObject, dt: number) {
var moveTime = dt - this.dt;
//Init values
var t = dt / 1000;
var g = 9.81;
var m = shape.getMass();
var A = shape.getCrossSection();
var C = shape.getDragCoefficient();
var rho = this.airDensity;
//Calculate speed
var v = Math.sqrt((2 * m * g) / (rho * A * C)) * tanh(t * Math.sqrt((g * rho * C * A) / (2 * m)));
//Move object
var dy = (v * moveTime) / 3;
shape.nudge(0, dy);
//Prepare for next loop
this.dt = dt;
}
}
Data
To prove my point, I thought it would be a good idea to have three balls of more or less the same size and shape - hence the golf ball, squash ball and ping pong ball. They have a mass of 46 g, 24 g and 2 g, respectively.
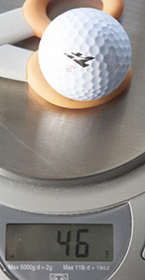
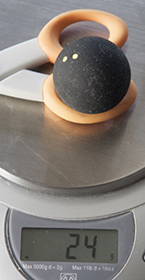
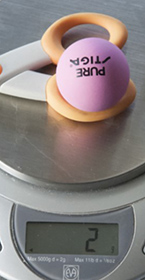
Using the formula for fall velocity in vacuum, we can calculate the time it takes to fall a certain distance. For our simulation, let's use 14.115 m.
The time t it takes for an object to fall distance d in vacuum is calculated with the following formula.
Using that formula with our data, it should take 1696 ms for an object to fall 14.115 m in vacuum. We'll use that as a starting point for our simulation, assuming that objects affected by air resistance at least won't fall faster than that.
Simulation
Time to run the numbers!
Wow! That looks nothing like the basket ball and medicine ball example. These three little rascals are at completely different heights after just 1.7 seconds. It looks like the lightest ball is the slowest, while the heaviest ball is the fastest. According to theory and my simulation, this would be correct, but according to the guy on YouTube, it should not be possible!
So... What if I were to...
Check the facts - for real!
I live in a flat, fourth floor. I have a balcony. The height from the edge of the balcony to the ground below is - guess what - 14.115 m. See where this is going?
Balcony setup
To deploy three balls more or less simultaneously, I duct-taped three cups to a cardboard piece, taped to a mortarboard, taped to a piassava, held in place by a big weight. The cups are attached to a fishing line that can be operated from the ground below.
The operator on the ground below would be me - with a camera. From the ground, the setup looks like this.
Pulling the fishing line gently, the balls fell out of their cups. It looks like the angle of the cups gave the pink ping pong ball a head start.
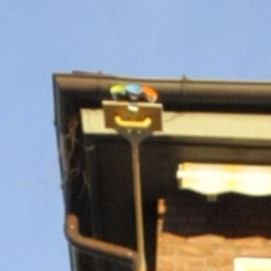
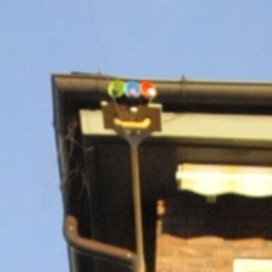
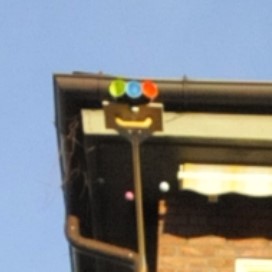
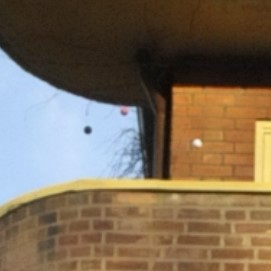
Here are the two last pictures of them before they hit the ground.
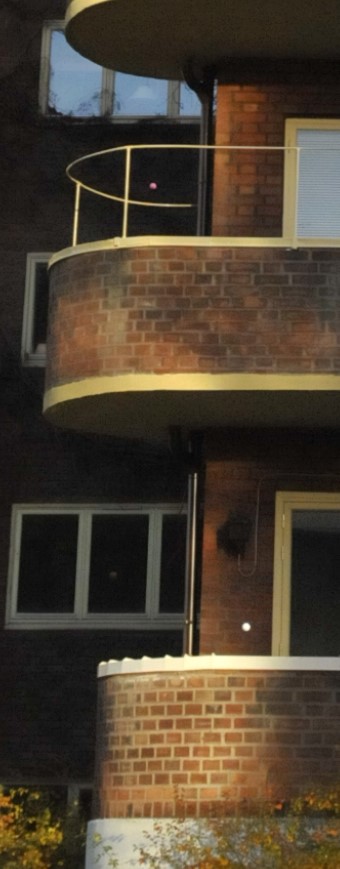
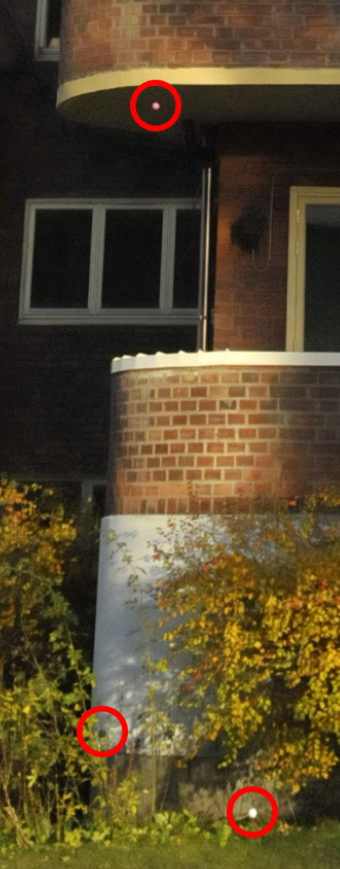
Compare the picture on the right with my simulation.
Conclusion(s)
Heavier objects DO fall faster, given similar shape and size. Larger parachutes will of course compensate.
TypeScript is brilliant for creating structured applications in JavaScript. Classes, interfaces, type safety, IntelliSense etc. saved me a lot of time when I built the simulator.
That YouTube guy is actually wrong - his arguments are only valid in a vacuum.
Peter and Tom claim it was a misunderstanding, and we all agree that drag affects fall velocity. We're all friends now. Water under the bridge.
You can check out the simulator yourself. Press Init, specify duration, and press Start simulation
P.S. There's another guy on YouTube
Brian Cox from BBC visited the world's largest vacuum chamber a while back, just to compare dropping a bowling ball and a feather in air and in vacuum. It is a very good demonstration of the physics presented in this post.